How to set up Google OAuth with Next.js using Next-Auth
This guide runs through the full setup of OAuth with Google as the identity provider. This will let your users log in with Google.
Google Cloud Console
The best way to get through the console is to watch the video above.
Google Cloud Platform
Google Cloud Platform lets you build, deploy, and scale applications, websites, and services on the same infrastructure as Google.
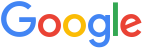
You will need to create your OAuth consent screen and then create the OAuth Credentials. With those, you can setup Next-Auth.
Session Helper
I like to create a helper function that will return the user in a session from the server.
import { User, getServerSession } from 'next-auth'
export const getUserSession = async (): Promise<User> => {
const authUserSession = await getServerSession()
return authUserSession?.user
}
If you need to pass through additional data, you'll need to use the same session
method to ensure that data is correctly passed through:
import { User, getServerSession } from 'next-auth'
export const session = async ({ session, token }: any) => {
session.user.id = token.id
session.user.tenant = token.tenant
return session
}
export const getUserSession = async (): Promise<User> => {
const authUserSession = await getServerSession({
callbacks: {
session
}
})
if (!authUserSession) throw new Error('unauthorized')
return authUserSession.user
}
The full example makes it a little more clear.
Code
Become a free member to get the complete source code below.
examples/next/oauth-google at main · a-bit-of-saas/examples
Example code for learning. Contribute to a-bit-of-saas/examples development by creating an account on GitHub.